Programming
HomeProgramming
Programming has always been a fun and intriguing activity! Here are my programming blog posts based on day-to-day experience and adventures with the programming languages and toolset.
Also includes a curated list of beginner-friendly tutorials, examples as well as real-world challenges and problem-solving methods. Currently includes Java, Maven, Hibernate, VB, etc., and still growing.


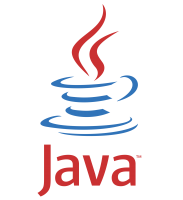
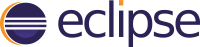


- Advertisement -
- Advertisement -
- Advertisement -
JAVA
HIBERNATE
VBSCRIPT
MAVEN
PROGRAMMING POSTS
Introduction to Apache Maven
1. What is Maven When you write a software application, there are many steps in it like adding the necessary JAR files, compiling the source code, running unit tests, creating the output jar/war file, etc. This is where Maven comes into play, it automates the build process, dependency management, testing,...
Getting Started With Hibernate
Introduction Hibernate is a framework which allows you to access database tables from Java code. Before Hibernate came into existence, JDBC was widely used. JDBC is an API to access a relational database from a java program. JDBC basically allows you to execute SQL statements from Java code, so any...
How To Sort List in Java
Introduction A List is an interface in the Java collection framework. It can be used to store objects. Programmers often encounter scenarios where they need to sort a List. There are several ways you can achieve this. In the next few sections, I will be going over each method in...
How To Convert String To Date in Java
Introduction There are often scenarios in programming, where you will need to convert a date in String format to an actual Date object. For example, such a scenario may occur when the date is read from an end user via a UI and sent to the back-end code. In this...
- Advertisement -
How To Concatenate Strings in Java
Introduction In Java, a String is a sequence of characters. There are often programming situations where you will need to concatenate Strings. There are several ways in which you can achieve this. In the next few sections, I will be going over each method in detail. Using + Operator The + operator...
Setting Max/Min Digits For Decimal Numbers With Java DecimalFormat
The DecimalFormat class offers the following four such methods which can be used to easily set the maximum and/or minimum digits for decimal numbers. As shown in the below table, two of these methods can set max/min digits for integer part while the other two methods can do the...
Rounding Decimal Number With Java DecimalFormat
The DecimalFormat class has a method called setRoundingMode() which can be used for setting the rounding mode for DecimalFormat object. The setRoundingMode() accepts RoundingMode class object as the parameter. You can pass any of the Enum constants available in the RoundingMode class as parameter to the setRoundingMode() method to...
Format Decimal Numbers with Grouping Separator
The DecimalFormat class has a method called setGroupingSize() which sets how many digits of the integer part to group. Groups are separated by the grouping separator. Hence you can use setGroupingSize() method to change the default group digits number as you need. The following example demonstrates setting grouping separator for...
- Advertisement -
Java DecimalFormat Class
When you need to format decimal numbers, such as taking three or two decimal places for a number, showing only the integer part of a number, etc. such scenarios can be tackled with java.text.DecimalFormatclass, which can help you to format numbers using your specified pattern. The java.text.DecimalFormat class is used...
Format Decimal Numbers using Strings Within Pattern
As mentioned in earlier posts, the java.text.DecimalFormat class is used to format decimal numbers via predefined patterns specified as String. Apart from the decimal separator, grouping separator, currency separator etc., It is also possible to mix String literals within the pattern. The following example demonstrates embedding String literals within pattern: b8c17d2e8b2af4b43df92c35de7031e8 The above...
Format Decimal Numbers Using Format Symbols
You can customize which symbols are used as decimal separator, grouping separator, currency seperator etc. using a DecimalFormatSymbols instance together with java.text.DecimalFormat class. The DecimalFormatSymbols instance can be passed to java.text.DecimalFormat constructor followed by the call to format() method of java.text.DecimalFormat to achieve the desired decimal separator,...
CODE GISTS
CronTab Basic Commands With Examples
Cron is a time-based job scheduler in Unix-like operating systems, which is used to automate repetitive tasks. It is a daemon (a background process)...
Concatenate Strings Using String.join()
Java 8 has added a static join method to the String class. This concatenates Strings using a delimiter. Performance wise, it is similar to...
Remove Duplicates from List Using LinkedHashSet
Another implementation of the Set interface is LinkedHashSet. LinkedHashSet maintains the order of elements and helps to overcome the HashSet limitation. The following code...
Convert List to Array Using For-Loop
The simplest way to convert a List to an array is to use a for-loop. The following code snippet demonstrates this method. First, the code...